Introduction
Table borders play a crucial role in presenting tabular data in a clear and visually appealing way. For example, they are essential when highlighting key financial metrics in a report, ensuring that important figures are easy to distinguish and understand. Whether you are creating a simple data table or designing a complex layout, understanding how to add and customize table borders using HTML and CSS can significantly impact the overall aesthetics and usability of your webpage. In this guide, we will explore different methods to add and style table borders, ranging from basic HTML attributes to advanced CSS techniques that provide greater flexibility and control over the design.
Adding effective borders helps distinguish between sections of data, create a well-organized layout, and make your tables stand out. Borders add definition and improve readability, making it easier for users to process the information presented. Additionally, styling borders can help guide the viewer's focus to key parts of the table, which is particularly helpful for enhancing the user's experience. Let’s look at various approaches for adding and customizing table borders so you can find the best solution for your design needs.
Adding Basic Table Borders Using HTML
One of the simplest ways to add a border to a table is by using the border attribute in HTML. This approach is easy to implement, especially for quick projects or when working with older HTML versions. Here’s an example:
<table border="1">
<tr>
<th>Item</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Apples</td>
<td>10</td>
<td>$5.00</td>
</tr>
<tr>
<td>Oranges</td>
<td>5</td>
<td>$3.50</td>
</tr>
</table>
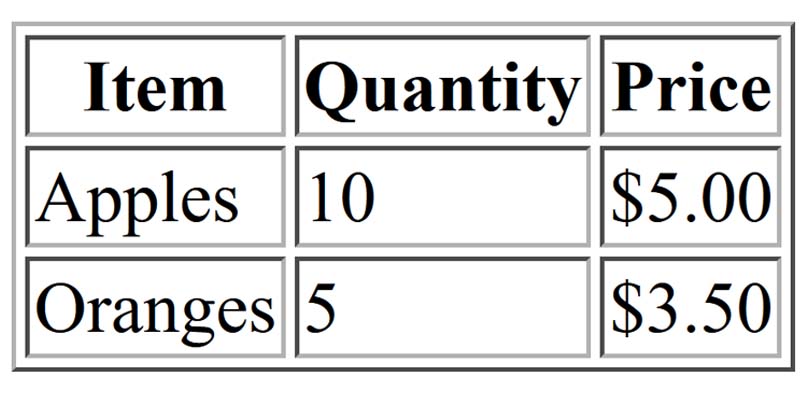
In the example above, the border="1" attribute adds a simple border around the table and its cells. However, this approach is outdated and lacks the flexibility required for modern web design. The static borders cannot be customized effectively to adjust color, style, or padding, which makes it challenging to maintain a consistent visual style across different tables on a webpage. To create more visually appealing and adaptable table borders, CSS is the preferred method. Using CSS also ensures consistency across different tables, which is crucial for maintaining a cohesive website design.
Adding and Customizing Table Borders Using CSS
CSS provides significantly more control over the appearance of table borders compared to basic HTML attributes. By using CSS, you can adjust the border's thickness, color, and style for both the entire table and individual cells. Here’s an example:
<style>
table {
border: 2px solid black;
border-collapse: collapse;
}
th, td {
border: 1px solid gray;
padding: 10px;
}
</style>
<table>
<tr>
<th>Item</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Bananas</td>
<td>8</td>
<td>$4.00</td>
</tr>
<tr>
<td>Grapes</td>
<td>15</td>
<td>$6.50</td>
</tr>
</table>
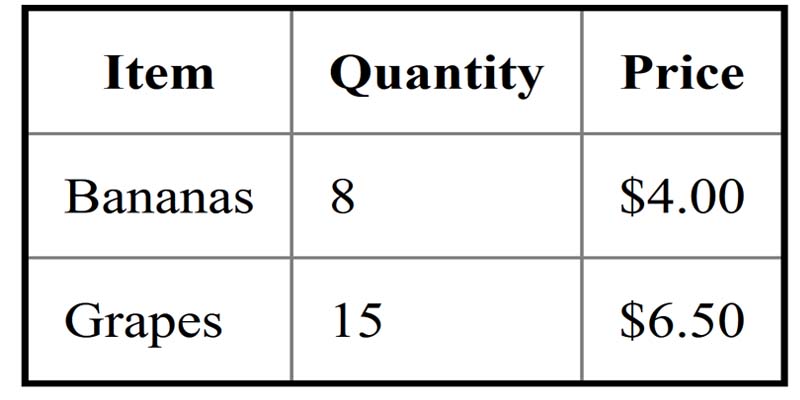
In this example, CSS is used to add a 2px solid black border around the table, while each cell (<th> and <td>) has a 1px solid gray border. The border-collapse: collapse; property ensures that adjacent borders merge into a single line, resulting in a cleaner appearance. The use of padding also creates space between the content and the cell borders, enhancing readability. CSS offers versatility in adjusting the visual style without altering the HTML structure, which makes it easier to maintain the code over time. This separation is especially beneficial in larger projects where design changes are frequent, as it allows developers to update the visual elements without modifying the underlying HTML, thereby reducing the risk of introducing errors and ensuring consistency across the site.
CSS allows you to experiment with different colors, thicknesses, and styles of borders, which makes it ideal for projects that require a polished and professional look. It also enables you to use consistent styles throughout your tables, giving your website a cohesive aesthetic that is both functional and visually appealing.
Styling Specific Sides of the Border
CSS also allows you to customize the borders on specific sides of a table or table cell. For example, you may want a thicker border at the top or use different colors for each side of the cell:
<style>
table {
border-top: 3px solid blue;
border-bottom: 3px solid green;
}
th, td {
border-left: 2px solid orange;
border-right: 2px solid red;
}
</style>
<table>
<tr>
<th>Item</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Pears</td>
<td>6</td>
<td>$3.75</td>
</tr>
<tr>
<td>Peaches</td>
<td>12</td>
<td>$7.20</td>
</tr>
</table>
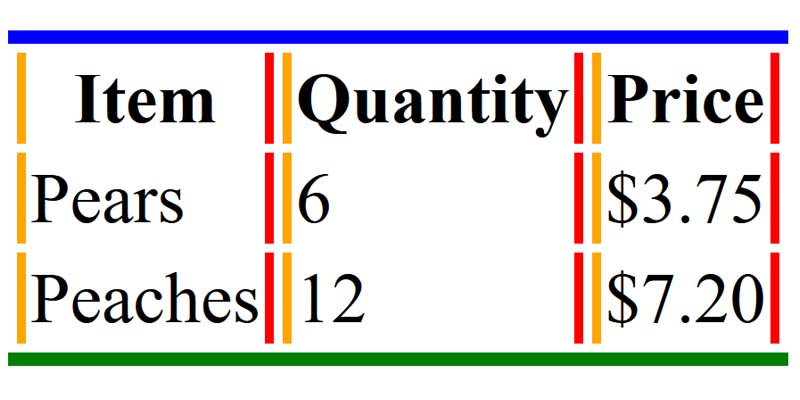
This approach allows you to create visually interesting tables by applying different styles to specific sides of the borders. For instance, applying a thicker border at the top of the table can make the header stand out, while using different colored borders for the sides of cells can help emphasize certain sections of the table.
CSS flexibility allows you to add emphasis where needed, such as highlighting a specific row or column. This is particularly useful for drawing attention to critical information, like totals in financial reports. In addition to using borders for highlighting, you can pair them with other design elements like background colors to create a more dynamic and visually engaging presentation of data.
Using CSS Classes for Reusability
When working with multiple tables on the same webpage, it’s best to use CSS classes to maintain consistency and avoid repetition. CSS classes let you apply the same border styles across different tables without having to rewrite the same code:
<style>
.table-border {
border: 2px solid #8b0000; /* DarkRed color */
border-collapse: collapse;
}
.cell-border {
border: 1px solid #2e8b57; /* SeaGreen color */
padding: 8px;
}
</style>
<table class="table-border">
<tr>
<th class="cell-border">Item</th>
<th class="cell-border">Quantity</th>
<th class="cell-border">Price</th>
</tr>
<tr>
<td class="cell-border">Cherries</td>
<td>20</td>
<td>$10.00</td>
</tr>
<tr>
<td class="cell-border">Blueberries</td>
<td>30</td>
<td>$15.00</td>
</tr>
</table>
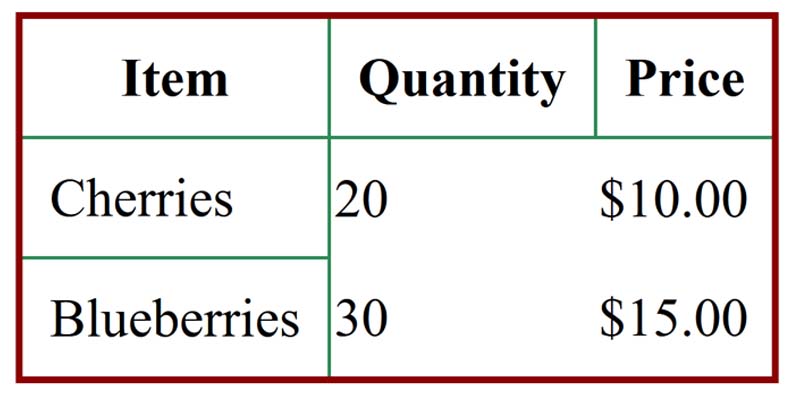
Using classes like .table-border and .cell-border makes your HTML code more organized and your CSS easier to maintain. For example, if you need to update the border color or style across multiple tables, you can do so by simply editing the class definition. This saves time and ensures that all tables remain consistent without needing to manually modify each one. You can make updates to the style in one place, and all tables using the class will reflect those changes.
This approach also ensures consistent styling across multiple tables, which is particularly important for creating a professional and cohesive design throughout the website. Uniformity is especially beneficial for business websites or data-heavy dashboards. CSS classes allow you to efficiently manage and maintain styling as your project scales, ensuring that design updates are applied consistently across all relevant elements.
Advanced Border Styles with CSS
CSS allows you to get creative with table borders by applying different styles, such as dashed, dotted, or double borders. This flexibility is useful when you want your tables to have a visually distinct style:
<style>
table {
border: 4px double #4b0082; /* Indigo color */
border-collapse: separate;
border-spacing: 5px;
}
th, td {
border: 2px dashed #ffa500; /* Orange color */
padding: 12px;
}
</style>
<table>
<tr>
<th>Item</th>
<th>Quantity</th>
<th>Price</th>
</tr>
<tr>
<td>Strawberries</td>
<td>25</td>
<td>$12.00</td>
</tr>
<tr>
<td>Raspberries</td>
<td>18</td>
<td>$9.50</td>
</tr>
</table>
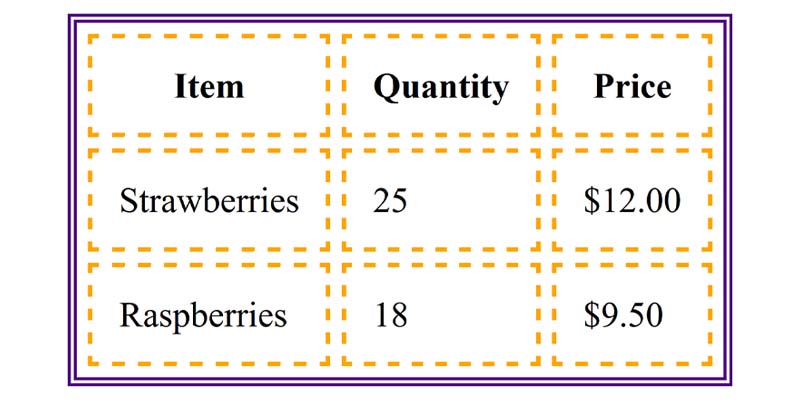
In this example, the table uses a double border style, while the cells use a dashed border style, making the table more visually engaging. The border-spacing property adds extra space between the cells, improving readability. Advanced styles let you make your table a focal point of the page, especially when you want to create a unique, branded look. Choosing the right combination of border styles, colors, and spacing can give your table a distinctive appearance that complements your website's overall theme.
These advanced border styles can help convey a particular brand image or differentiate specific types of data. For example, advanced border styles can be used to differentiate between sections of an invoice, such as using a double border for the total amount due to make it stand out, or a dotted border for optional items or additional notes. Such design choices help add visual hierarchy to your tables and make it easier for users to interpret the data presented.
Combining Background Colors and Borders
Another effective way to enhance the visual appeal of your tables is by combining background colors with borders. Adding background colors to header cells or rows can differentiate sections of the table, which helps organize information and makes it easier to interpret:
<style>
.custom-table {
border: 3px solid #2f4f4f; /* DarkSlateGray color */
border-collapse: collapse;
}
.header-cell {
background-color: #dcdcdc; /* Gainsboro color */
font-weight: bold;
border: 1px solid #000000; /* Black color */
padding: 10px;
}
.data-cell {
background-color: #f5f5f5; /* WhiteSmoke color */
border: 1px solid #696969; /* DimGray color */
padding: 10px;
}
</style>
<table class="custom-table">
<tr>
<th class="header-cell">Item</th>
<th class="header-cell">Quantity</th>
<th class="header-cell">Price</th>
</tr>
<tr>
<td class="data-cell">Mangoes</td>
<td>12</td>
<td>$10.00</td>
</tr>
<tr>
<td class="data-cell">Pineapples</td>
<td>7</td>
<td>$14.00</td>
</tr>
</table>
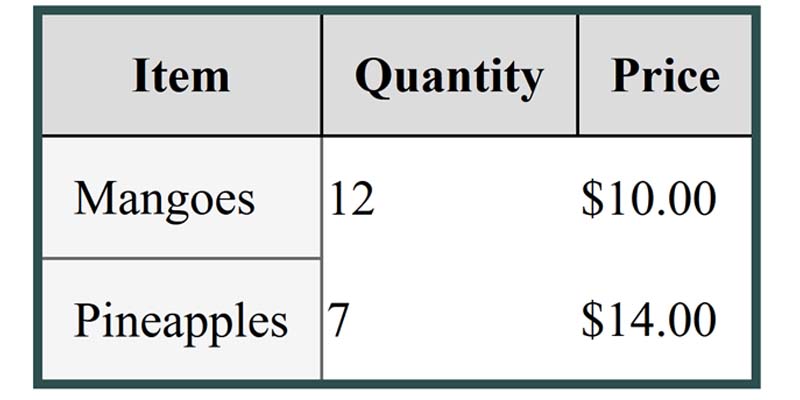
In this example, the combination of background colors and borders helps separate the header row from the data rows, providing a polished and organized look. This makes the table more visually appealing and easier to read, which is particularly useful when dealing with large sets of data.
Adding background colors along with borders is especially helpful for creating contrast between different parts of the table, guiding the viewer's eye to the most critical information. You can also use background colors to indicate various categories or data types, making your tables more intuitive and informative.
Conclusion
Adding and customizing table borders using HTML and CSS can significantly enhance the presentation of your data. While the HTML border attribute is a simple way to add borders, using CSS provides far greater flexibility and control, enabling you to create professional and visually appealing tables. By using CSS properties like border, border-collapse, and border-spacing, as well as reusable CSS classes, you can design tables that effectively present data and improve user experience.
Furthermore, combining borders with background colors and employing advanced CSS techniques can elevate your tables, ensuring they are both functional and attractive. A well-designed table contributes to better user engagement and effectively conveys information, making your website more user-friendly and professional. By leveraging these CSS tools, you can create data presentations that are not only informative but also visually striking, ultimately enhancing the value your website provides to users.