If you're reading this article, you've likely decided to start learning programming. Given the growth and potential in this field, you may have just made one of the most important decisions of your life. Congratulations, and welcome to the journey of becoming a programmer!
One of the first things you'll learn when diving into programming is syntax. If you've heard the term before, you might be wondering, "What exactly is syntax in programming, and why is it important?" Let's explore this in the simplest way possible.
What is Syntax in Programming?
In the world of programming, syntax refers to the rules that define the structure of a programming language. Just like in human languages, syntax in programming is about how you write and arrange the symbols, punctuation, and keywords that make up your code. These rules help computers understand what you want them to do.
Think of syntax like grammar in a spoken language. If you make a grammatical mistake, it can make a sentence confusing. Similarly, incorrect syntax in programming means the computer won't understand what you've written, and this will usually result in what's called a syntax error.
For example, in English, the sentence "goes she home" is incorrect because it doesn't follow the rules of grammar. In programming, if the syntax is wrong, the code won’t run, and the computer might show an error message that means, "I don't understand what you're trying to tell me."
Why Is Syntax Important?
Without correct syntax, code cannot be understood by the computer. Here’s why paying attention to syntax is critical:
Correctness: Computers are very literal. Unlike humans, they cannot guess what you mean if you make a small mistake. Incorrect syntax will prevent the program from running.
Readability: Syntax also helps make your code readable to other people, including your future self. A well-structured syntax makes it easy to understand what the code does.
Consistency: Using consistent syntax means that your code is easier to maintain and modify in the future.
Examples of Basic Syntax Rules
Every programming language has its own unique syntax. Let’s explore the basic syntax through an example: how to print the message "Hello, World!". Below are examples in ten different popular programming languages:
Syntax in C# Programming Examples
C# is a modern, object-oriented language developed by Microsoft. Its syntax is similar to C++ and Java, and it's known for its simplicity and versatility.
using System;
class Program
{
static void Main()
{
Console.WriteLine("Hello, World!");
}
}
Syntax in Python Programming
Python is a high-level, interpreted language known for its easy-to-read syntax. It is often recommended for beginners due to its straightforward syntax rules.
print("Hello, World!")
Syntax in Java Programming
Java is a class-based, object-oriented programming language that is designed to have as few implementation dependencies as possible.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Syntax in JavaScript Programming
JavaScript is a lightweight, interpreted programming language used primarily for adding interactive features to web pages.
console.log("Hello, World!");
Syntax in C++ Programming
C++ is an extension of the C programming language, and it includes object-oriented features, making it versatile for various programming tasks.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Syntax in PHP Programming
PHP is a popular scripting language that is especially suited to web development. It is fast, flexible, and pragmatic.
<?php
echo "Hello, World!";
?>
Syntax in Swift Programming
Swift is a powerful and intuitive programming language for macOS, iOS, watchOS, and tvOS, developed by Apple.
print("Hello, World!")
Syntax in Ruby Programming
Ruby is an open-source, dynamic programming language with a focus on simplicity and productivity.
puts "Hello, World!"
Syntax in Go Programming
Go, also known as Golang, is a statically typed, compiled programming language designed at Google for simplicity and reliability.
package main
import "fmt"
func main() {
fmt.Println("Hello, World!")
}
Syntax in Kotlin Programming
Kotlin is a statically typed programming language that runs on the Java Virtual Machine (JVM) and is fully interoperable with Java.
fun main() {
println("Hello, World!")
}
Common Syntax Elements Across Languages
No matter what language you are learning, there are common syntax elements you will come across:
Keywords: These are reserved words with special meanings, like if
, else
, for
, or while
.
Operators: Symbols like +
, -
, *
, or /
are used to perform operations on data.
Punctuation: Characters such as ;
or {}
help structure the code.
Variables: These are names that store data, such as age
, name
, or isValid
.
Learning to Handle Syntax Errors
When you start writing code, you will definitely encounter syntax errors. Syntax errors happen when the code you write does not follow the rules of the programming language. Unlike humans, computers cannot make educated guesses about what you mean—the instructions must be exact.
The good news is that most programming tools will help you find and fix syntax errors. When an error happens, take a deep breath, look at the message, and correct the mistake. Syntax errors are part of the learning process, and every programmer, even the experienced ones, makes them from time to time.
The Importance of Learning Syntax for Faster Programming Progress
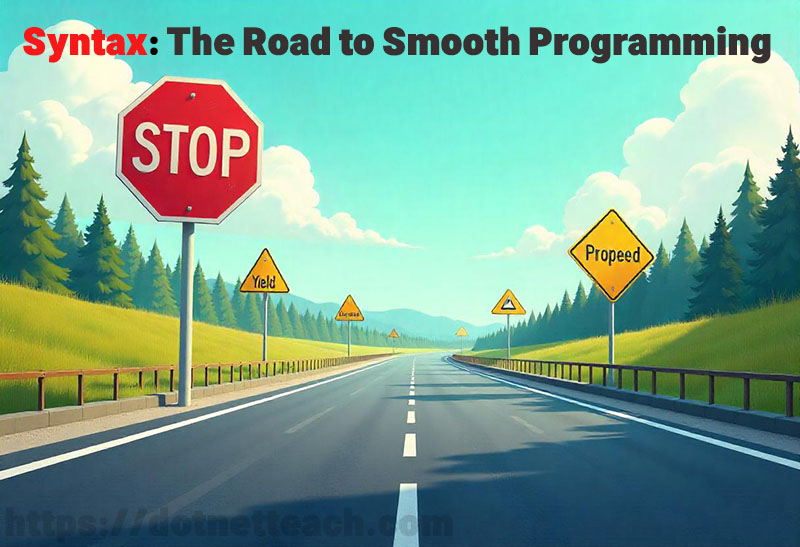
Understanding syntax is crucial for learning programming quickly and effectively. Just as learning proper grammar helps someone communicate better in a new spoken language, mastering syntax helps you communicate your intentions clearly to the computer. When you have a solid grasp of syntax, you can write code more accurately and with fewer mistakes, which means you spend less time debugging and more time building.
Imagine syntax as the rules of the road. If you know when to stop, yield, and proceed, your journey will be smoother and faster. Likewise, knowing the correct syntax helps you avoid errors and navigate through learning programming concepts more efficiently. A strong understanding of syntax empowers you to learn other programming concepts, like loops and conditionals, at a much faster pace.
Conclusion
Syntax is like the glue that holds programming together. It allows you to communicate your intentions clearly to the computer so that your code runs smoothly. By learning the syntax of your chosen programming language, you are taking the first step toward becoming a proficient programmer.
If you want to learn more about programming concepts and dive deeper into C# or other languages, make sure to check out our website at dotnetteach.com. It's packed with tutorials and resources to help you grow as a programmer.
Happy coding!