Hey there! Welcome to the world of C#. If you've just started programming, you've made a fantastic choice by diving into C#. It's a powerful and versatile language, but don’t worry, we’ll take it step by step together. Today, we'll explore some basic C# syntax in a way that’s simple and easy to understand. Think of this as a conversation where I’m here to help you understand how C# works—like a guide leading you through a new and exciting place. Ready? Let’s go!
If you're not familiar with syntax yet, I recommend checking out our previous article titled Syntax in Programming. It's a helpful resource that explains these concepts more deeply.
Your First Program: "Hello World!"
The first program most people write when they learn any programming language is the famous "Hello World!". It’s like your first "Hello" to the world of coding! Here's what it looks like in C#:
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
Don’t worry if this looks like a lot of new words. Let’s break it down, piece by piece. I promise it will all make sense!
Breaking Down the Code
1. using System;
This line tells C# that we want to use a specific set of tools from the System namespace. Think of it like adding a toolbox to your project that has all the necessary tools to do simple things like printing messages to the screen.
2. namespace HelloWorld
A namespace is like a folder for organizing your code. It keeps everything neat and avoids confusion, especially when your projects get bigger. Here, our namespace is called HelloWorld
.
3. class Program
A class is like a blueprint that describes what your code can do. Every bit of code in C# needs to be inside a class. Here, we have a class called Program
. Don’t worry about what this does just yet—for now, just know that everything starts with a class.
4. static void Main(string[] args)
The Main method is where your program starts running. You can think of it as the entry point—kind of like the "Start" button on a machine. Whenever you run your program, C# looks for Main
to begin.
For now, don't get caught up in all the words like static
, void
, or string[] args
. Just know that every C# program needs this line to get going.
5. Console.WriteLine("Hello, World!");
Here comes the fun part! This line tells the computer to print "Hello, World!" on the screen.
Console
is a part of the System toolbox, and it allows you to interact with the user.
WriteLine
is a command that means "print this and move to the next line."
Pretty cool, right? It’s our way of saying "Hey, computer, display this text!"
If you are using .NET 6 or later, you don't need to write the namespace or the Main method. You can simply write the code like this:
Console.WriteLine("Hello, World!");
This will output:
.png)
Play Around With It!
Now that you understand the pieces, try playing around with the code. Change "Hello, World!"
to something else like "Hello, [Your Name]!"
. When you run it, you’ll see your message appear on the screen.
For example:
Console.WriteLine("Hello, Jennifer!");
This will output:
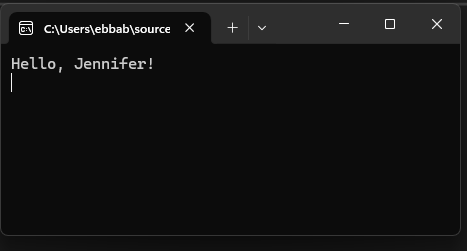
Key Concepts of C# Syntax
Let’s go over some important C# syntax rules. Don’t worry if you don’t memorize them all right away—just practice, and soon it will all feel natural.
Semicolons (;
): Every line of code that tells the computer to do something must end with a semicolon. It’s like the period at the end of a sentence.
Curly Braces ({}
): These are used to group things together. When you see {}
in C#, think of them as bookends that say, "Everything in between belongs together." For example, the Main
method has its own curly braces to contain its code.
Case Sensitivity: C# is case-sensitive. That means Main
is different from main
. Always pay attention to how things are spelled.
Example: Making Your Own Simple Program
Let’s write another program. This time, let’s make it ask for your name and then greet you. Note that this program uses the top-level statements feature introduced in .NET 6 and later versions, which means we don’t need to write the Main
method inside a Program
class—these parts are already written and available for you! Here’s the code:
Console.WriteLine("What is your name?");
string name = Console.ReadLine();
Console.WriteLine("Hello, " + name + "! Nice to meet you!");
This will output:
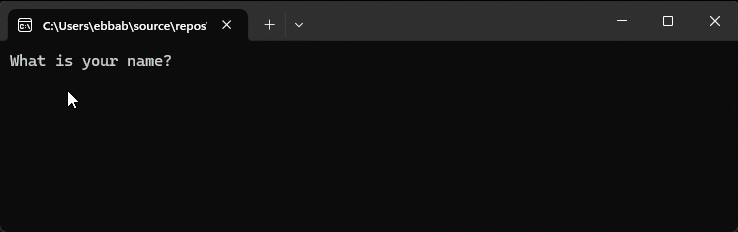
Explanation
Console.ReadLine();
This command waits for the user to type something and press Enter. Here, it’s used to get your name.
string name = Console.ReadLine();
This line saves whatever the user types into a variable called name
. Think of a variable like a little box that stores information.
A variable is like a container that stores information for you. Imagine it like a labeled box where you can put something inside and retrieve it later whenever you need it. Variables help us store data, such as numbers or text, which we can use throughout our programs.
In C#, you declare a variable by specifying its type (what kind of information it will hold) and giving it a name. Let’s look at an example of how to create and use a string variable in C#:
string greeting = "Hello, world!";
Console.WriteLine(greeting);
string
: This tells C# that the variable will hold text.
greeting
: This is the name of the variable. You can name it anything you want, as long as it follows the rules of C# naming.
= "Hello, world!"
: This assigns the text "Hello, world!" to the variable greeting
.
When you run the code, it will output:
Hello, world!
You can change the value of greeting
and use it again to display different messages! called name
. Think of a variable like a little box that stores information
Console.WriteLine("Hello, " + name + "! Nice to meet you!");
This line greets the user using whatever they typed in. The +
symbol is used to join bits of text together.
Example code in visual studio
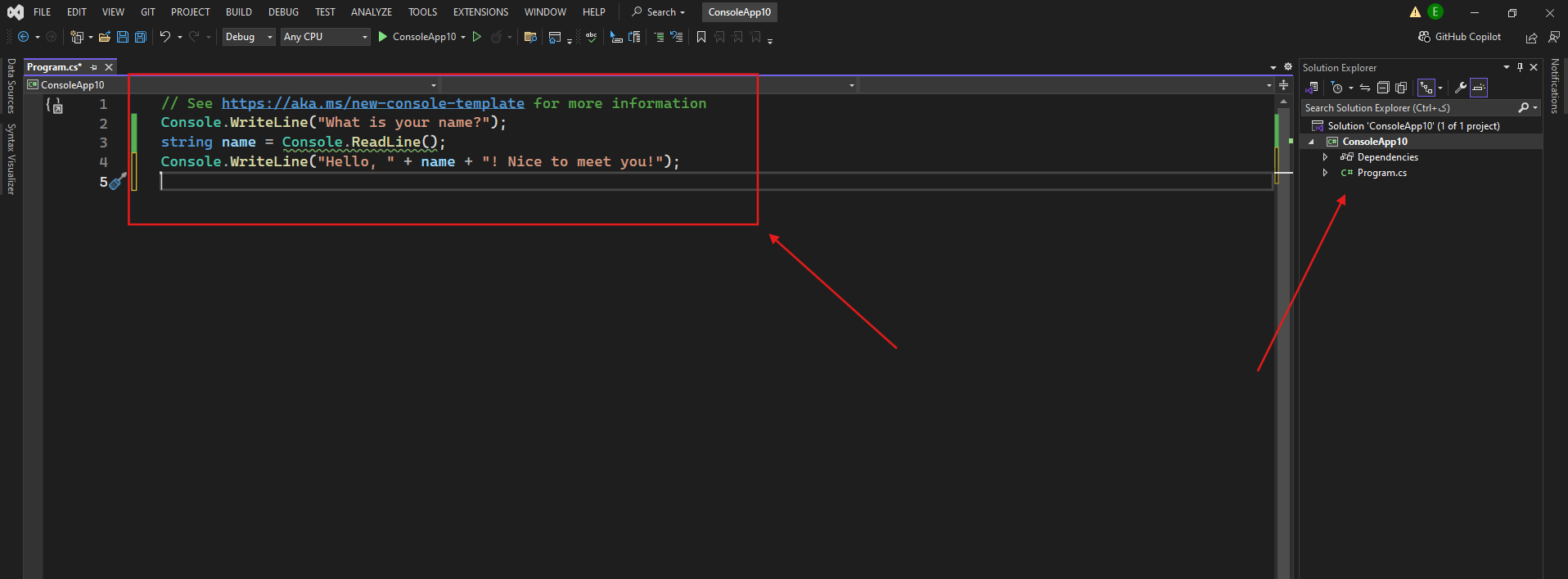
Give It a Try!
Try typing and running this code. Notice how it interacts with you. Go ahead and experiment! What happens if you change "Hello, "
to "Hey, "
or "Hi there, "
? Little changes like this make your program feel more personal.
Keep Practicing!
Programming is like learning a new language—the more you practice, the better you get. Don’t worry if things feel confusing at first. Every programmer started exactly where you are right now, and each step forward is progress. Keep trying different things, and don’t be afraid to make mistakes. Mistakes are just part of the journey.
I hope this guide has helped you take your first steps with C# syntax. Remember, we’re just scratching the surface, and there’s so much more to discover. You’ve got this—let’s keep learning together!
Happy coding! 😊